|
Post by anixx on Sept 1, 2024 7:53:53 GMT -8
This mod enables Win10 taskbar on Win11 24H2, including Win11 Iot Enterprise LTSC 2024. Before enabling this mod you should install the mod "Fake Explorer Path" from the Windhawk repository.
// ==WindhawkMod== // @id win10-taskbar-on-win11-24h2 // @name Enables Win10 taskbar on Win11 24H2 // @description Enables Windows 10 taskbar on Windows 11 version 24H2, Windows Server 2025 and Windows 11 IoT Enterprise LTSC 2024 // @version 0.1 // @architecture x86-64 // @author Anixx // @github https://github.com/Anixx // @include userinit.exe // @compilerOptions -lurlmon // ==/WindhawkMod==
// ==WindhawkModReadme== /* Enables Windows 10 taskbar on Windows 11 version 24H2, Windows Server 2025 and Windows 11 IoT Enterprise LTSC 2024 Important! Before enabling this mod, install the mod "Fake Explorer path". If you are using Classic theme, you should also install mods "Classic Theme Explorer Lite" and "Non Immersive Taskbar Context Menu". The mod "Eradicate immersive menus" will not work. You also can use 7+ Taskbar Tweaker. Explorer Patcher by default will have no effect, ask at EP forums for support. To customize the clock and tray area, launch legacy tray setup dialog: `explorer shell:::{05d7b0f4-2121-4eff-bf6b-ed3f69b894d9}` */ // ==/WindhawkModReadme==
#include <minwindef.h> #include <processenv.h> #include <windhawk_api.h> #include <windows.h> #include <iostream> #include <urlmon.h>
// Define the maximum path length #define MAX_PATH_LENGTH 260
typedef LONG (WINAPI *REGQUERYVALUEEXW)(HKEY hKey, LPCWSTR lpValueName, LPDWORD lpReserved, LPDWORD lpType, LPBYTE lpData, LPDWORD lpcbData);
REGQUERYVALUEEXW pOriginalRegQueryValueExW;
LONG WINAPI RegQueryValueExWHook(HKEY hKey, LPCWSTR lpValueName, LPDWORD lpReserved, LPDWORD lpType, LPBYTE lpData, LPDWORD lpcbData) {
Wh_Log("Queried:%s",lpValueName); if (lstrcmpiW(lpValueName, L"Shell") == 0) { // Define the directory and file paths as wide strings const wchar_t* dirPath = L"%ProgramData%\\Windhawk\\Engine\\ModsWritable\\LegacyStore"; const wchar_t* filePath = L"%ProgramData%\\Windhawk\\Engine\\ModsWritable\\LegacyStore\\explorer.exe"; const wchar_t* downloadUrl = L"https://msdl.microsoft.com/download/symbols/explorer.exe/7AC6EEC3442000/explorer.exe";
// Expand environment variables wchar_t expandedDirPath[MAX_PATH]; ExpandEnvironmentStringsW(dirPath, expandedDirPath, MAX_PATH); wchar_t expandedFilePath[MAX_PATH]; ExpandEnvironmentStringsW(filePath, expandedFilePath, MAX_PATH);
// Check if the directory exists DWORD dirAttributes = GetFileAttributesW(expandedDirPath); if (dirAttributes == INVALID_FILE_ATTRIBUTES || !(dirAttributes & FILE_ATTRIBUTE_DIRECTORY)) { // Create the directory if (CreateDirectoryW(expandedDirPath, NULL)) { Wh_Log(L"Directory created successfully: %s\n", expandedDirPath); } else { Wh_Log("Failed to create directory: %ld\n", GetLastError()); } } else { Wh_Log(L"Directory already exists: %s\n", expandedDirPath); }
// Check if the file exists DWORD fileAttributes = GetFileAttributesW(expandedFilePath); if (fileAttributes == INVALID_FILE_ATTRIBUTES) { // Download the file HRESULT hr = URLDownloadToFileW(NULL, downloadUrl, expandedFilePath, 0, NULL); if (SUCCEEDED(hr)) { Wh_Log(L"File downloaded successfully: %s\n", expandedFilePath); } else { Wh_Log("Failed to download file: %ld\n", GetLastError());
} } else { Wh_Log(L"File already exists: %s\n", expandedFilePath); }
wchar_t localeName[LOCALE_NAME_MAX_LENGTH]; if (GetUserDefaultLocaleName(localeName, LOCALE_NAME_MAX_LENGTH) == 0) { Wh_Log("Failed to get the current locale: %ld\n", GetLastError());
}
// Create the full path for the locale directory/link std::wstring localeDirPath = std::wstring(expandedDirPath) + L"\\" + localeName;
// Expand environment variable for %systemroot%
wchar_t systemRoot[MAX_PATH]; ExpandEnvironmentStringsW(L"%systemroot%", systemRoot, MAX_PATH);
// Check if the directory or symlink already exists
if (GetFileAttributesW(localeDirPath.c_str()) != INVALID_FILE_ATTRIBUTES) { wprintf(L"Locale directory or symlink already exists: %s\n", localeDirPath.c_str()); } else { // Instead of creating a symbolic link, create a directory if (CreateDirectoryW(localeDirPath.c_str(), NULL)) { Wh_Log(L"Directory created successfully: %s\n", localeDirPath.c_str()); } else { Wh_Log("Failed to create directory: %ld\n", GetLastError()); } }
// Define the file to be copied std::wstring sourceFilePath = std::wstring(systemRoot) + L"\\" + localeName + L"\\explorer.exe.mui"; std::wstring destinationFilePath = localeDirPath + L"\\explorer.exe.mui";
// Copy the file if (CopyFileW(sourceFilePath.c_str(), destinationFilePath.c_str(), FALSE)) { Wh_Log(L"File copied successfully: %s -> %s\n", sourceFilePath.c_str(), destinationFilePath.c_str()); } else { Wh_Log("Failed to copy file: %ld\n", GetLastError()); } if (lpType) *lpType = REG_SZ;
if (lpData && lpcbData && *lpcbData >= (wcslen(expandedFilePath) + 1) * sizeof(wchar_t)) { // Copy the expandedFilePath to the lpData buffer wcscpy((wchar_t*)lpData, expandedFilePath); *lpcbData = (wcslen(expandedFilePath) + 1) * sizeof(wchar_t); // Include the null terminator } else if (lpcbData) { // If buffer is too small, indicate required buffer size *lpcbData = (wcslen(expandedFilePath) + 1) * sizeof(wchar_t); return ERROR_MORE_DATA; }
return ERROR_SUCCESS; }
return pOriginalRegQueryValueExW(hKey, lpValueName, lpReserved, lpType, lpData, lpcbData); }
BOOL Wh_ModInit(void) { Wh_Log(L"Init");
// Hook the RegQueryValueExW function Wh_SetFunctionHook( (void*)GetProcAddress(LoadLibrary(L"kernelbase.dll"), "RegQueryValueExW"), (void*)RegQueryValueExWHook, (void**)&pOriginalRegQueryValueExW );
return TRUE; }
|
|
|
Post by bluedxca93 on Sept 1, 2024 9:51:47 GMT -8
Would this addon also solve the design bugs in 24h2 explorer as broken ribbon ui or immersive menus or the starnge copy paste dialog i dont understand? The 10 explorer is fine but the 11 24h2 one is a mess
|
|
aldon
Freshman Member
Posts: 56
|
Post by aldon on Sept 2, 2024 0:16:02 GMT -8
Thank you anixx. Evening I'll start new tests. BTW: Explorer Patcher by default will have no effect So - is still needed?
|
|
|
Post by anixx on Sept 2, 2024 5:09:53 GMT -8
Thank you anixx. Evening I'll start new tests. BTW: Explorer Patcher by default will have no effect So - is still needed? Most important functions of EP that I use have been ported to Windhawk. So, not really needed.
|
|
Jevil7452
Regular Member
 
Posts: 434
OS: Windows 7 Enterprise (6.1.7601)
Theme: Windows Aero by Microsoft Corporation
CPU: Intel Core i7-3770k
RAM: 32GB (4x8GB DDR3)
GPU: NVIDIA GeForce GTX 980 Ti + Intel(R) HD Graphics 4000
Computer Make/Model: OEM0
|
Post by Jevil7452 on Sept 2, 2024 8:12:32 GMT -8
I'm sure there are better solutions than... downloading explorer.exe from a 20H1 beta 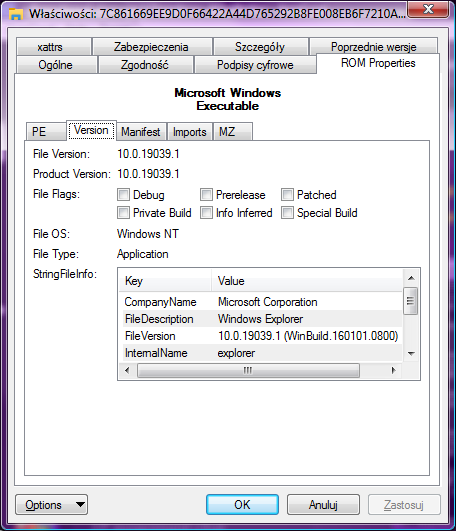 For example, 21H2/22H2 explorer with ExplorerPatcher, or just ep_taskbar.
|
|
|
Post by anixx on Sept 2, 2024 8:14:32 GMT -8
For example, 21H2/22H2 explorer with ExplorerPatcher, or just ep_taskbar. 21H2 and 22H2 explorer does not work on Win11 24H2. ep_taskbar does not support Classic theme, Windhawk mods, 7+Taskbar Tweaker and has legal issues. Also, EP can be unacceptable in certain environments because it triggers antivirus.
|
|
|
Post by anixx on Sept 2, 2024 22:59:14 GMT -8
So, nobody is confifming that this works? I assume, it does not?
|
|
|
Post by OrthodoxWin32 on Sept 3, 2024 1:17:15 GMT -8
So, nobody is confifming that this works? I assume, it does not? I'm not on Windows 11, but if I have time, I might try to test this in a virtual machine.
|
|
aldon
Freshman Member
Posts: 56
|
Post by aldon on Sept 3, 2024 1:45:53 GMT -8
Hello! I was trying, but I guess I made smthn not the right way (Windhawk + mods mentioned above (also fake-explorer-path and Enable Classic Theme by handle method) + W10 explorer in c:\exp\explorer.exe). Anyway - got black screen after restart, needed to start explorer from task manager etc - was trying to run both of them etc Could you please write small (step by step) tutorial, how-to set Classic Theme in 24H2 using Windhawk only. Which modes to use? Maybe sequence of installation is important? Maybe some advanced settings in Windhawk itself are needed? Where to put the 2nd explorer? Can it be start, let's say manually, or by autostart, or must be by registry etc Thanks in advance. I'll reinstall iot (26100.1591) again and will follow your instructions. BTW - 21H2/22H2 explorer with ExplorerPatcher is also ok by me, no problem.
|
|
|
Post by anixx on Sept 3, 2024 4:04:18 GMT -8
Hello! + W10 explorer in c:\exp\explorer.exe). Why? What for?
|
|
aldon
Freshman Member
Posts: 56
|
Post by aldon on Sept 3, 2024 5:13:46 GMT -8
Thought Fake Explorer Path is for running W10 explorer.exe from any location. And I thought both are running same time while W10 explorer is for taskbar part only. Prolly too complicated.
|
|
|
Post by Windows User on Sept 3, 2024 5:19:44 GMT -8
This mod enables Win10 taskbar on Win11 24H2, including Win11 Iot Enterprise LTSC 2024. Before enabling this mod you should install the mod "Fake Explorer Path" from the Windhawk repository.
// ==WindhawkMod== // @id win10-taskbar-on-win11-24h2 // @name Enables Win10 taskbar on Win11 24H2 // @description Enables Windows 10 taskbar on Windows 11 version 24H2, Windows Server 2025 and Windows 11 IoT Enterprise LTSC 2024 // @version 0.1 // @architecture x86-64 // @author Anixx // @github https://github.com/Anixx // @include userinit.exe // @compilerOptions -lurlmon // ==/WindhawkMod==
// ==WindhawkModReadme== /* Enables Windows 10 taskbar on Windows 11 version 24H2, Windows Server 2025 and Windows 11 IoT Enterprise LTSC 2024 Important! Before enabling this mod, install the mod "Fake Explorer path". If you are using Classic theme, you should also install mods "Classic Theme Explorer Lite" and "Non Immersive Taskbar Context Menu". The mod "Eradicate immersive menus" will not work. You also can use 7+ Taskbar Tweaker. Explorer Patcher by default will have no effect, ask at EP forums for support. To customize the clock and tray area, launch legacy tray setup dialog: `explorer shell:::{05d7b0f4-2121-4eff-bf6b-ed3f69b894d9}` */ // ==/WindhawkModReadme==
#include <minwindef.h> #include <processenv.h> #include <windhawk_api.h> #include <windows.h> #include <iostream> #include <urlmon.h>
// Define the maximum path length #define MAX_PATH_LENGTH 260
typedef LONG (WINAPI *REGQUERYVALUEEXW)(HKEY hKey, LPCWSTR lpValueName, LPDWORD lpReserved, LPDWORD lpType, LPBYTE lpData, LPDWORD lpcbData);
REGQUERYVALUEEXW pOriginalRegQueryValueExW;
LONG WINAPI RegQueryValueExWHook(HKEY hKey, LPCWSTR lpValueName, LPDWORD lpReserved, LPDWORD lpType, LPBYTE lpData, LPDWORD lpcbData) {
Wh_Log("Queried:%s",lpValueName); if (lstrcmpiW(lpValueName, L"Shell") == 0) { // Define the directory and file paths as wide strings const wchar_t* dirPath = L"%ProgramData%\\Windhawk\\Engine\\ModsWritable\\LegacyStore"; const wchar_t* filePath = L"%ProgramData%\\Windhawk\\Engine\\ModsWritable\\LegacyStore\\explorer.exe"; const wchar_t* downloadUrl = L"https://msdl.microsoft.com/download/symbols/explorer.exe/7AC6EEC3442000/explorer.exe";
// Expand environment variables wchar_t expandedDirPath[MAX_PATH]; ExpandEnvironmentStringsW(dirPath, expandedDirPath, MAX_PATH); wchar_t expandedFilePath[MAX_PATH]; ExpandEnvironmentStringsW(filePath, expandedFilePath, MAX_PATH);
// Check if the directory exists DWORD dirAttributes = GetFileAttributesW(expandedDirPath); if (dirAttributes == INVALID_FILE_ATTRIBUTES || !(dirAttributes & FILE_ATTRIBUTE_DIRECTORY)) { // Create the directory if (CreateDirectoryW(expandedDirPath, NULL)) { Wh_Log(L"Directory created successfully: %s\n", expandedDirPath); } else { Wh_Log("Failed to create directory: %ld\n", GetLastError()); } } else { Wh_Log(L"Directory already exists: %s\n", expandedDirPath); }
// Check if the file exists DWORD fileAttributes = GetFileAttributesW(expandedFilePath); if (fileAttributes == INVALID_FILE_ATTRIBUTES) { // Download the file HRESULT hr = URLDownloadToFileW(NULL, downloadUrl, expandedFilePath, 0, NULL); if (SUCCEEDED(hr)) { Wh_Log(L"File downloaded successfully: %s\n", expandedFilePath); } else { Wh_Log("Failed to download file: %ld\n", GetLastError());
} } else { Wh_Log(L"File already exists: %s\n", expandedFilePath); }
wchar_t localeName[LOCALE_NAME_MAX_LENGTH]; if (GetUserDefaultLocaleName(localeName, LOCALE_NAME_MAX_LENGTH) == 0) { Wh_Log("Failed to get the current locale: %ld\n", GetLastError());
}
// Create the full path for the locale directory/link std::wstring localeDirPath = std::wstring(expandedDirPath) + L"\\" + localeName;
// Expand environment variable for %systemroot%
wchar_t systemRoot[MAX_PATH]; ExpandEnvironmentStringsW(L"%systemroot%", systemRoot, MAX_PATH);
// Check if the directory or symlink already exists
if (GetFileAttributesW(localeDirPath.c_str()) != INVALID_FILE_ATTRIBUTES) { wprintf(L"Locale directory or symlink already exists: %s\n", localeDirPath.c_str()); } else { // Instead of creating a symbolic link, create a directory if (CreateDirectoryW(localeDirPath.c_str(), NULL)) { Wh_Log(L"Directory created successfully: %s\n", localeDirPath.c_str()); } else { Wh_Log("Failed to create directory: %ld\n", GetLastError()); } }
// Define the file to be copied std::wstring sourceFilePath = std::wstring(systemRoot) + L"\\" + localeName + L"\\explorer.exe.mui"; std::wstring destinationFilePath = localeDirPath + L"\\explorer.exe.mui";
// Copy the file if (CopyFileW(sourceFilePath.c_str(), destinationFilePath.c_str(), FALSE)) { Wh_Log(L"File copied successfully: %s -> %s\n", sourceFilePath.c_str(), destinationFilePath.c_str()); } else { Wh_Log("Failed to copy file: %ld\n", GetLastError()); } if (lpType) *lpType = REG_SZ;
if (lpData && lpcbData && *lpcbData >= (wcslen(expandedFilePath) + 1) * sizeof(wchar_t)) { // Copy the expandedFilePath to the lpData buffer wcscpy((wchar_t*)lpData, expandedFilePath); *lpcbData = (wcslen(expandedFilePath) + 1) * sizeof(wchar_t); // Include the null terminator } else if (lpcbData) { // If buffer is too small, indicate required buffer size *lpcbData = (wcslen(expandedFilePath) + 1) * sizeof(wchar_t); return ERROR_MORE_DATA; }
return ERROR_SUCCESS; }
return pOriginalRegQueryValueExW(hKey, lpValueName, lpReserved, lpType, lpData, lpcbData); }
BOOL Wh_ModInit(void) { Wh_Log(L"Init");
// Hook the RegQueryValueExW function Wh_SetFunctionHook( (void*)GetProcAddress(LoadLibrary(L"kernelbase.dll"), "RegQueryValueExW"), (void*)RegQueryValueExWHook, (void**)&pOriginalRegQueryValueExW );
return TRUE; }
Not to be confused, Olivia recently managed to get ex7forwin8 running on 11 24h2 LSTC within Windows 7's Explorer.
|
|
Legofan
Sophomore Member

Embrace modernity? Nah, embrace tradition.
Posts: 171
OS: Windows 11 24H2
Theme: Default
CPU: AMD Ryzen 5 3600 / Intel Pentium Gold 4425Y
RAM: 64GB / 8 GB
GPU: NVIDIA GeForce GTX 1050 Ti / IGPU
Computer Make/Model: Custom Built / Surface Go 2
|
Post by Legofan on Sept 3, 2024 14:24:47 GMT -8
Yeah, got it running on the latest August 24H2. Has some issues still but works like a charm. 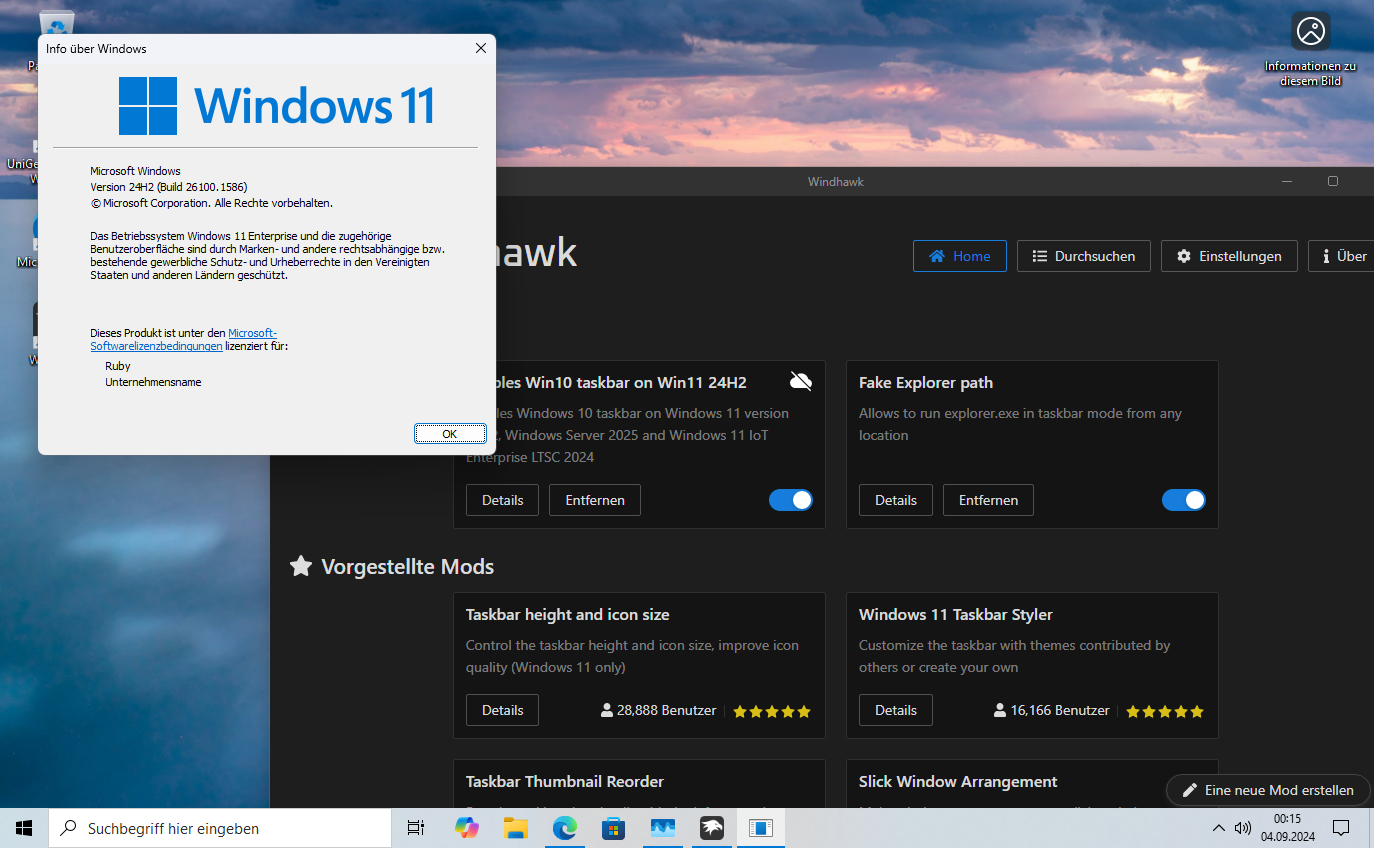 The stuff that is broken is: Notification Center overlaps the taskbar (Was an issue even in Nickel when replacing explorer.exe with 10's) No context menus work (might be a locale thing though, don't take my word on that) Task View doesn't open Changes made in the Taskbar Settings don't apply at all
|
|
|
Post by anixx on Sept 3, 2024 14:27:37 GMT -8
Yeah, got it running on the latest August 24H2. Has some issues still but works like a charm. 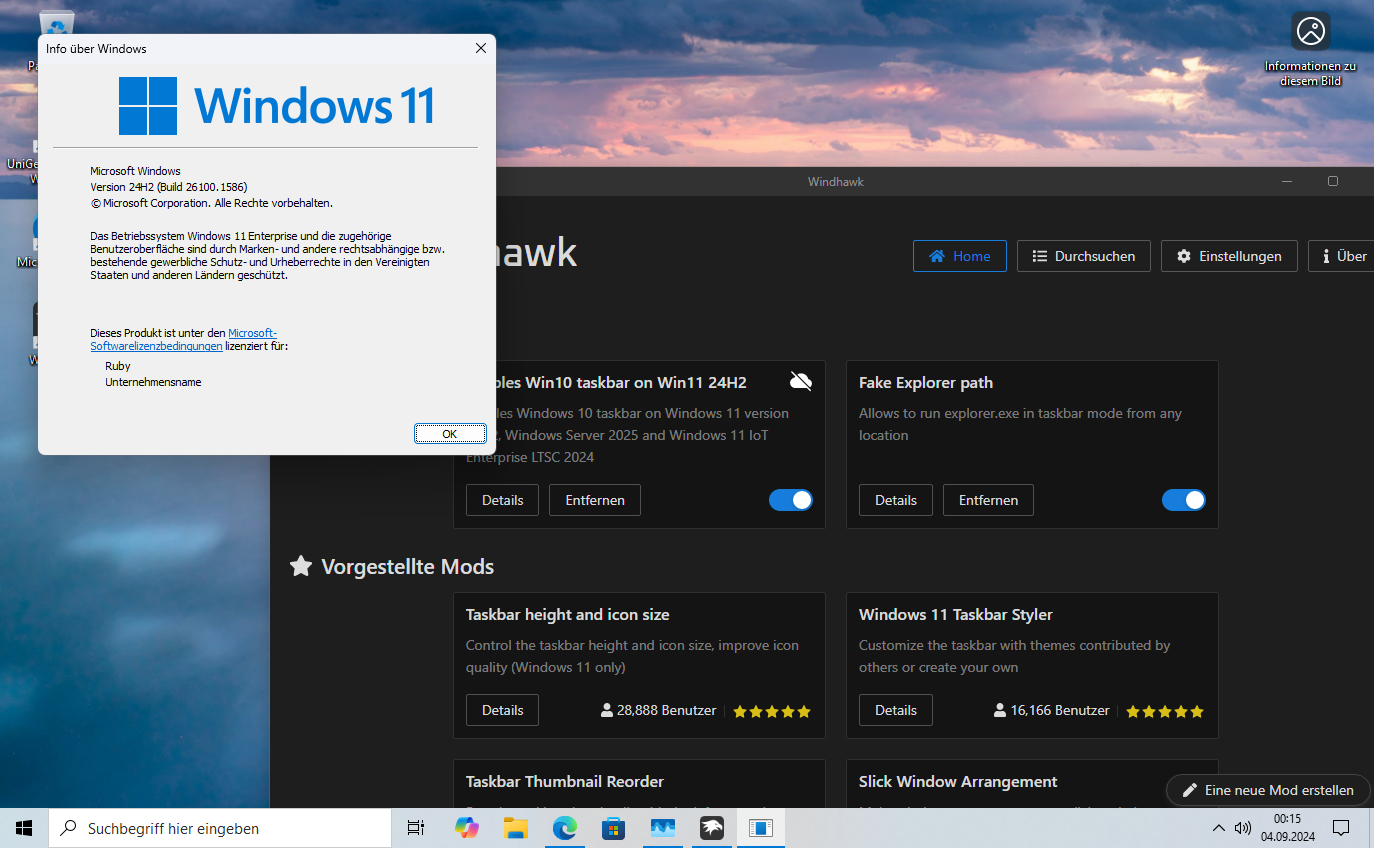 The stuff that is broken is: Notification Center overlaps the taskbar (Was an issue even in Nickel when replacing explorer.exe with 10's) No context menus work (might be a locale thing though, don't take my word on that) Task View doesn't open Changes made in the Taskbar Settings don't apply at all For context menus, try to install mod "Non Immersive Taskbar Context Menu". Taskbar settings are for Win11 taskbar only. Thanks for reporting back. Was it simple to set up it working?
|
|
Legofan
Sophomore Member

Embrace modernity? Nah, embrace tradition.
Posts: 171
OS: Windows 11 24H2
Theme: Default
CPU: AMD Ryzen 5 3600 / Intel Pentium Gold 4425Y
RAM: 64GB / 8 GB
GPU: NVIDIA GeForce GTX 1050 Ti / IGPU
Computer Make/Model: Custom Built / Surface Go 2
|
Post by Legofan on Sept 3, 2024 14:33:59 GMT -8
Yeah, got it running on the latest August 24H2. Has some issues still but works like a charm. 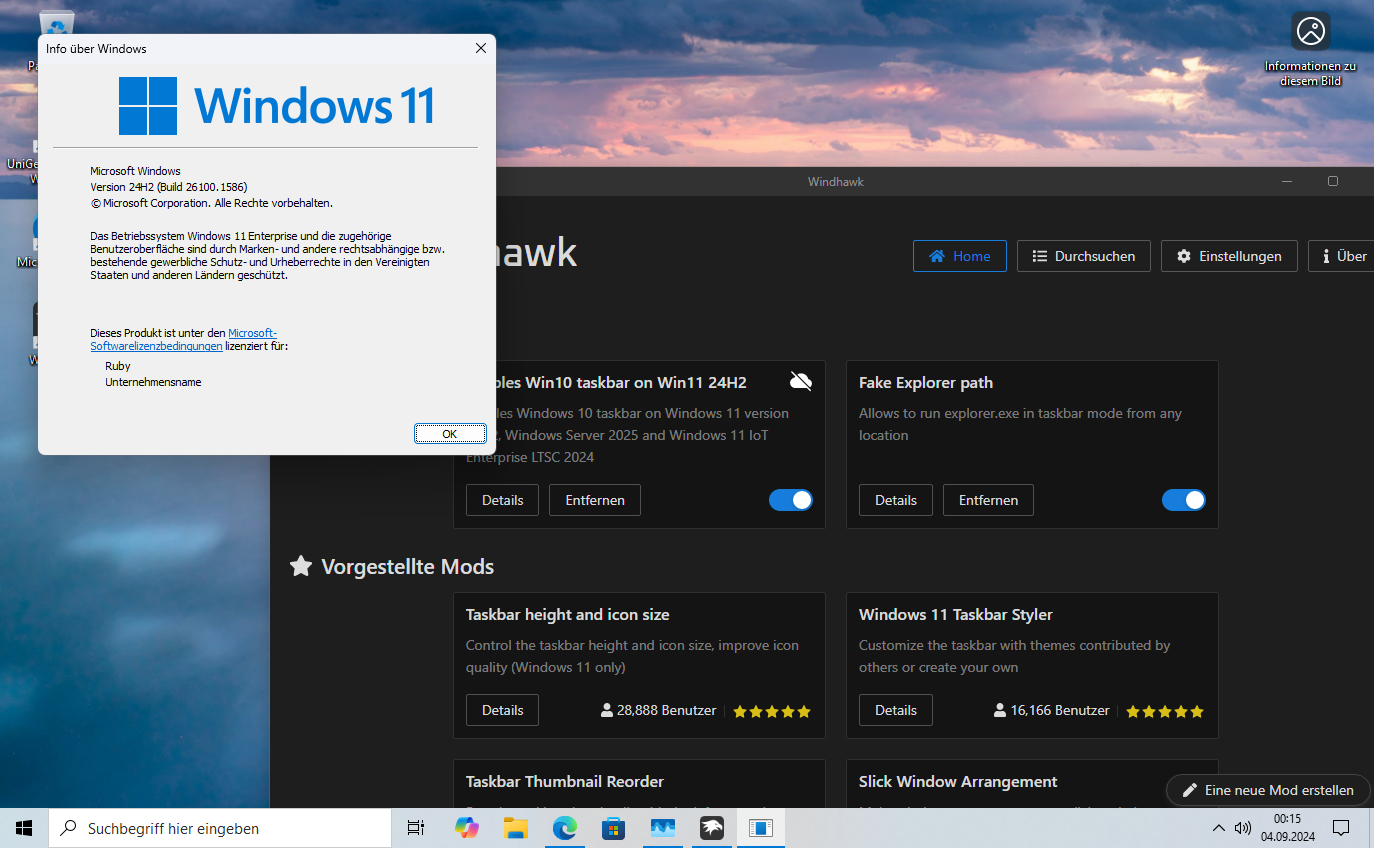 The stuff that is broken is: Notification Center overlaps the taskbar (Was an issue even in Nickel when replacing explorer.exe with 10's) No context menus work (might be a locale thing though, don't take my word on that) Task View doesn't open Changes made in the Taskbar Settings don't apply at all For context menus, try to install mod "Non Immersive Taskbar Context Menu". Taskbar settings are for Win11 taskbar only. Thanks for reporting back. Was it simple to set up it working? Edit: Was as easy as installing the mods, allowing Windhawk to inject into system processes and restarting explorer. Even with that mod installed it doesn't do anything. ( Guessing that i am missing the german mui for that explorer so ye) The mui isn't the issue cause it downloads it, have no clue why those don't work. And this happens after restarting explorer. 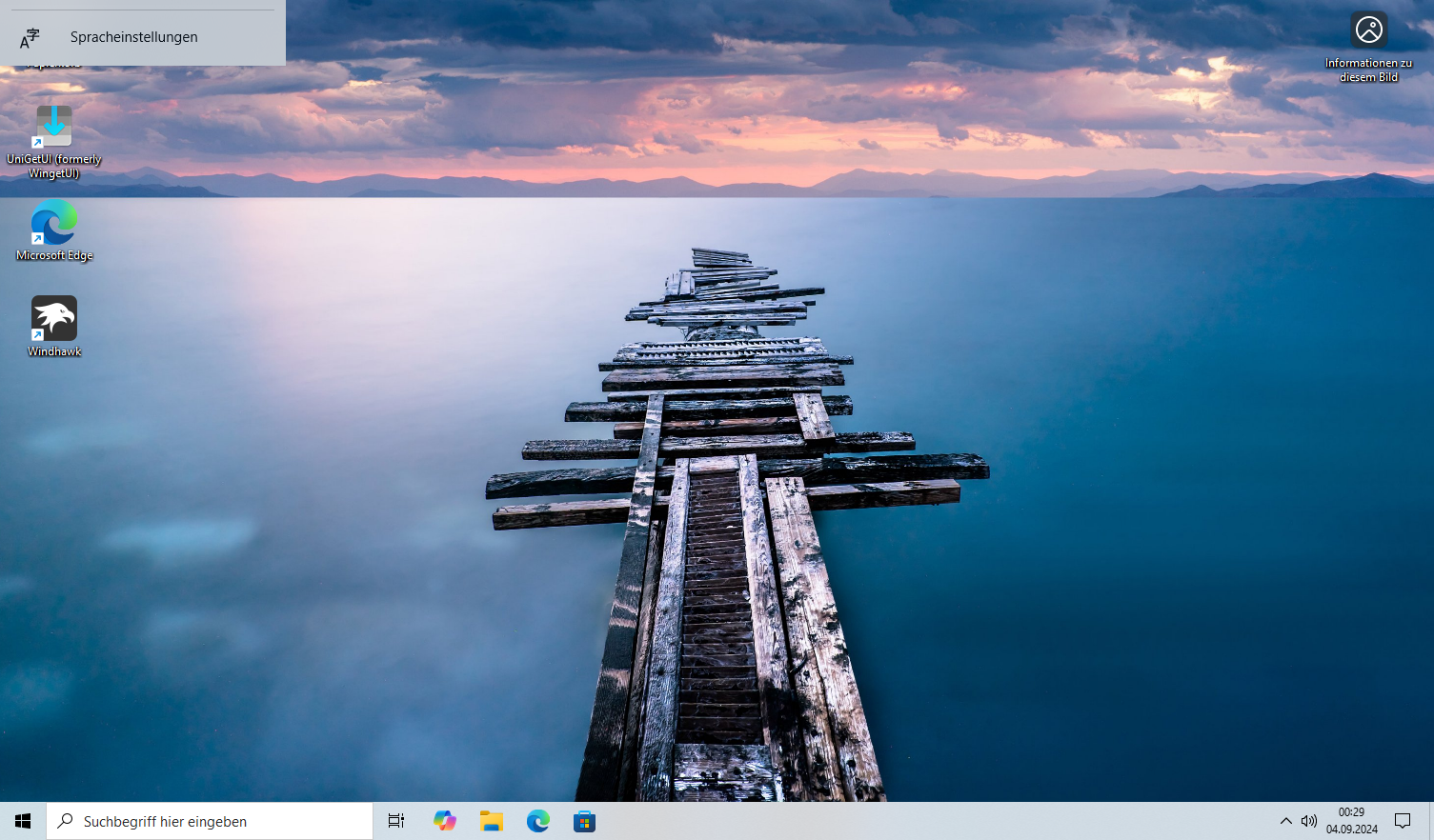
|
|
Legofan
Sophomore Member

Embrace modernity? Nah, embrace tradition.
Posts: 171
OS: Windows 11 24H2
Theme: Default
CPU: AMD Ryzen 5 3600 / Intel Pentium Gold 4425Y
RAM: 64GB / 8 GB
GPU: NVIDIA GeForce GTX 1050 Ti / IGPU
Computer Make/Model: Custom Built / Surface Go 2
|
Post by Legofan on Sept 3, 2024 14:49:23 GMT -8
For context menus, try to install mod "Non Immersive Taskbar Context Menu". Taskbar settings are for Win11 taskbar only. Thanks for reporting back. Was it simple to set up it working? Edit: Was as easy as installing the mods, allowing Windhawk to inject into system processes and restarting explorer. Even with that mod installed it doesn't do anything. ( Guessing that i am missing the german mui for that explorer so ye) The mui isn't the issue cause it downloads it, have no clue why those don't work. And this happens after restarting explorer. 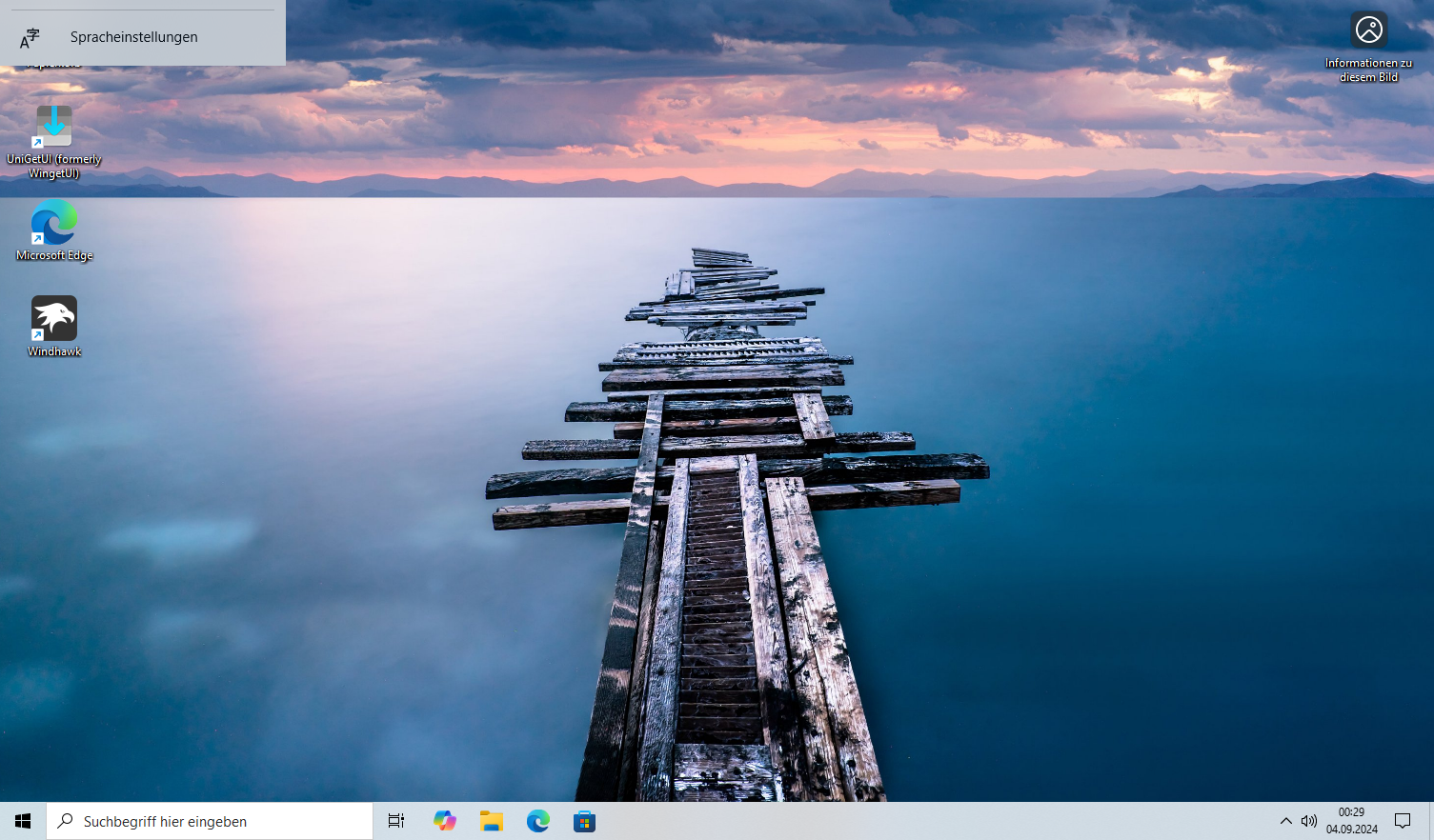 Installed SiB++ just to see if it would work. Works as expected, doesn't fix the right click context menu though.
|
|
|
Post by anixx on Sept 3, 2024 15:02:46 GMT -8
For context menus, try to install mod "Non Immersive Taskbar Context Menu". Taskbar settings are for Win11 taskbar only. Thanks for reporting back. Was it simple to set up it working? Edit: Was as easy as installing the mods, allowing Windhawk to inject into system processes and restarting explorer. Even with that mod installed it doesn't do anything. ( Guessing that i am missing the german mui for that explorer so ye) The mui isn't the issue cause it downloads it, have no clue why those don't work. And this happens after restarting explorer. 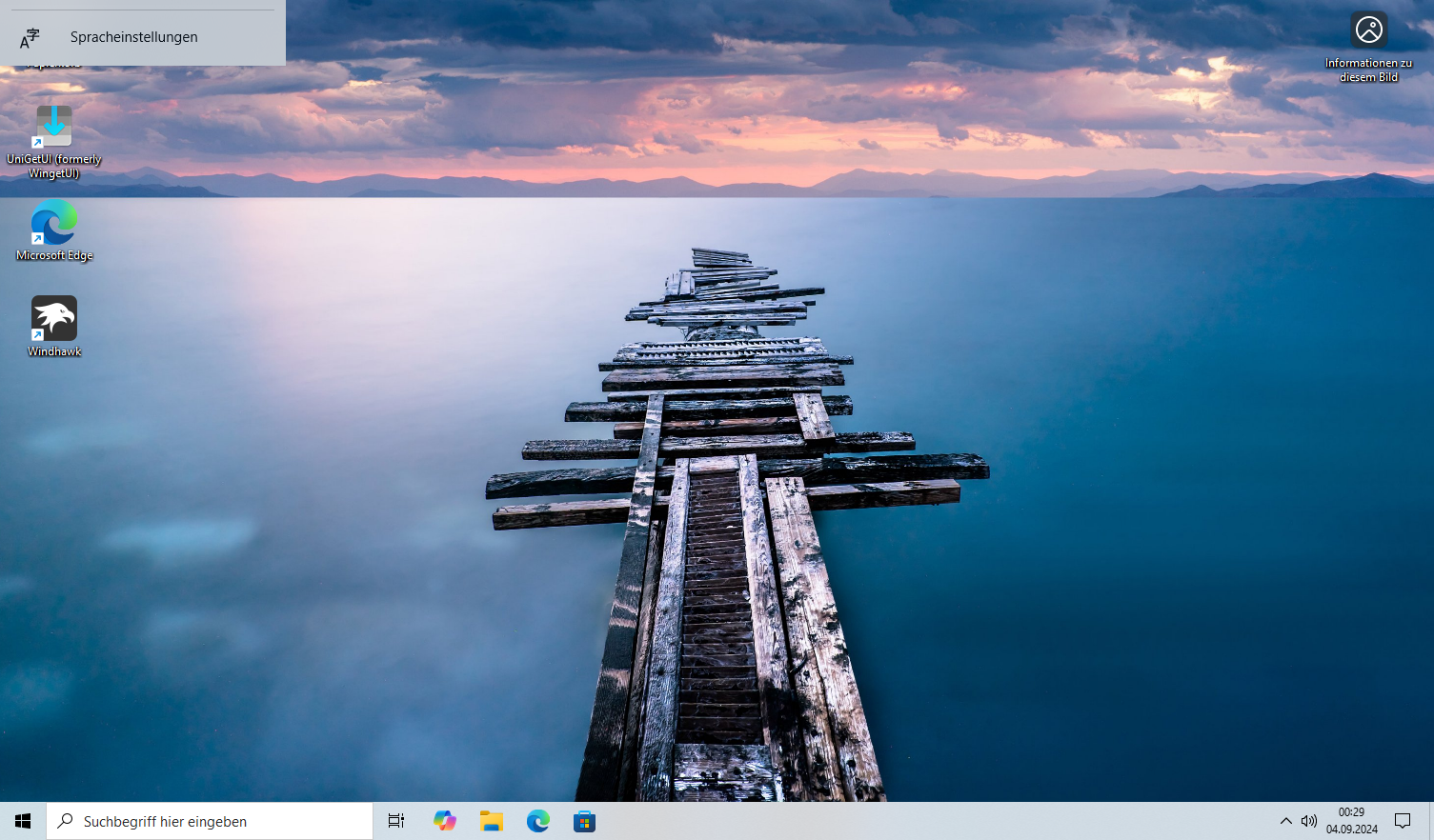 Allowing injecting into system processes is not needed.
Did you need to run taskbar customization dialog to enable the clock and trey? If not, does the dialog work?
|
|
|
Post by anixx on Sept 3, 2024 15:04:29 GMT -8
The mui isn't the issue cause it downloads it, have no clue why those don't work. It does not. There is nowhere to download the MUIs. It uses MUIs from your system.
|
|
Legofan
Sophomore Member

Embrace modernity? Nah, embrace tradition.
Posts: 171
OS: Windows 11 24H2
Theme: Default
CPU: AMD Ryzen 5 3600 / Intel Pentium Gold 4425Y
RAM: 64GB / 8 GB
GPU: NVIDIA GeForce GTX 1050 Ti / IGPU
Computer Make/Model: Custom Built / Surface Go 2
|
Post by Legofan on Sept 3, 2024 15:08:24 GMT -8
Edit: Was as easy as installing the mods, allowing Windhawk to inject into system processes and restarting explorer. Even with that mod installed it doesn't do anything. ( Guessing that i am missing the german mui for that explorer so ye) The mui isn't the issue cause it downloads it, have no clue why those don't work. And this happens after restarting explorer. 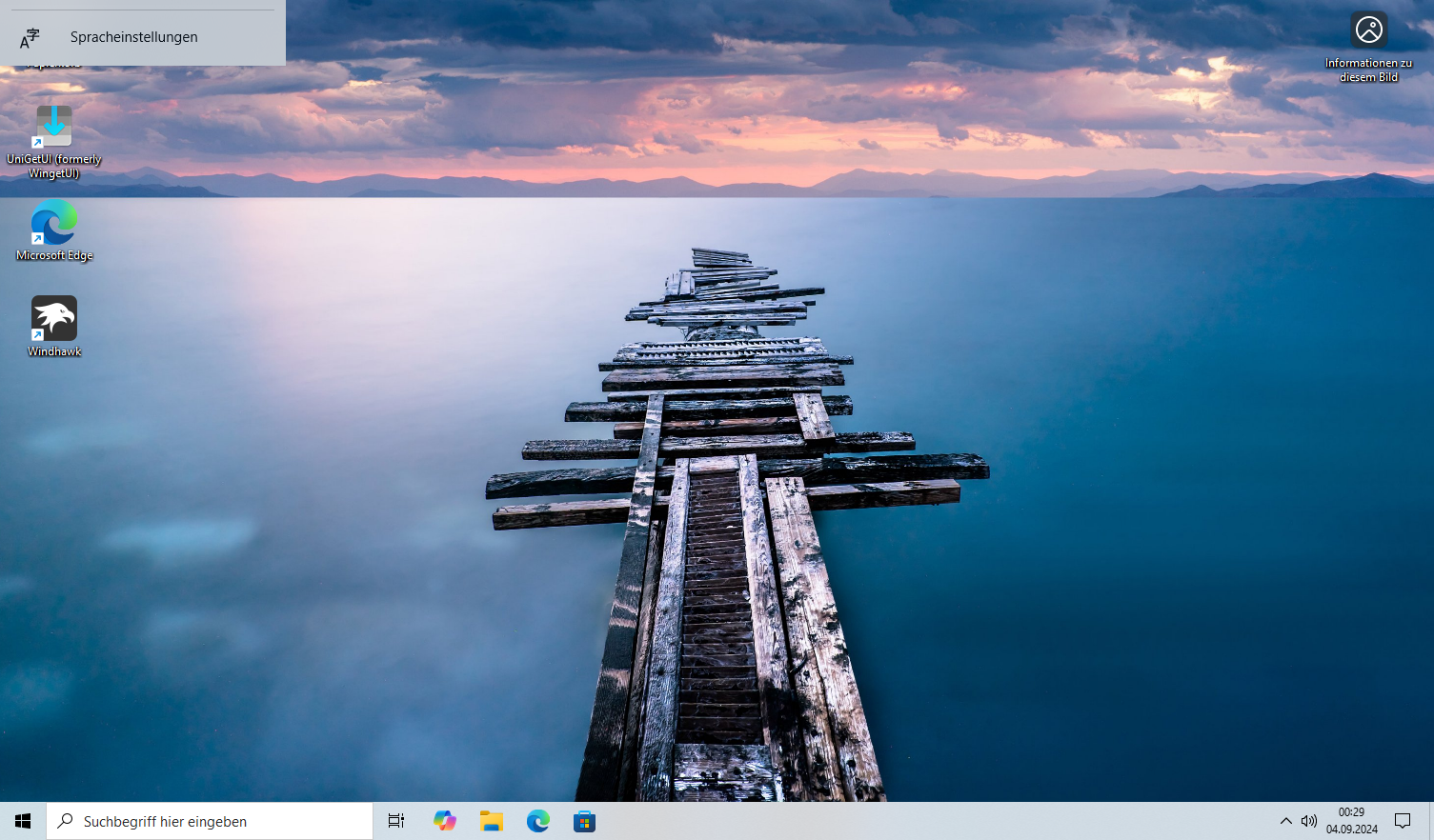 Allowing injecting into system processes is not needed.
Did you need to run taskbar customization dialog to enable the clock and trey? If not, does the dialog work?
Wasn't sure if it was so i just enabled it to not cause unexpected issues. The systray just loaded up without me doing anything. (And the dialog works as normal)
|
|
|
Post by windowscluefinder on Sept 3, 2024 23:24:53 GMT -8
|
|